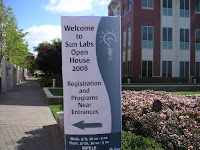
Today is the first day of Sun Labs Open House 2008 and I'm here in Menlo Park to participate in this event. I worked at Sun Labs last June for about 2 weeks to learn and create Sun SPOT demos, so my main purpose here is still for Sun SPOT, however, there were several cutting edge technologies other than it. So I'd like to introduce them as far as I can.
I heard some interesting JVM topics in the morning, but they are all internal-only sessions, so omit the details here. In the afternoon, I attended the following sessions:
- Sun Small Programmable Object Technology (SPOT)
- Project Live* [live star]
- Project Squawk
and watched some demos. Here is a summary:
Sun Small Programmable Object Technology (SPOT)This is a session to explain Sun SPOT overview & in-the-field including some demos. The agenda is divided into some topics: introduction, hardware, squawk, networking, security, solarium, emulator, in the field and hands on labs.
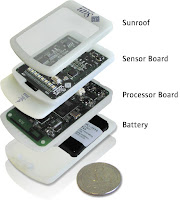
Sun SPOT device has three layers: battery, processor board with radio and sensor board. Processor board alone acts as a base-station that is connected to PC via USB to communicate with other Sun SPOT. And users can program the device entirely in Java using standard Java tools and/or IDE like
NetBeans.
Here is Sun SPOT processor board (what is so called "eSPOT") spec:
- 180 MHz 32 bit ARM920T core processor (Atmel AT91RM9200)
- 512K RAM/4M Flash PROM
- 2.4GHz 802.15.4 Radio Transceiver (TI CC2420)
- USB interface - mini-b connector
- Real Time Clock and Power Management Processor (Atmega88)
- 3.6V rechargeable 750 mAh lithium-ion battery
- 30 pin high density interboard connector
- “Java on the metal” - No OS. Squawk VM runs on the bare metal
eDemoboard (sensor board) spec is:
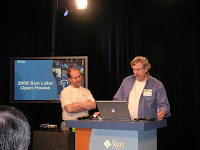
- 2G/6G 3-axis accelerometer
- Light sensor
- Temp sensor
- 2 push buttons
- 8 RGB 24 bit LEDS, power/control LED
- 6 analog inputs readable by a 12 bit ADC
- 5 General Purpose I/O pins (GPIO) and 4 high current output pins w/ Atmega88 IO processor
You can even make your own Sun SPOT hardware: eDemoboard is just a sample impl. shipped with Sun SPOT developer's kit (see the detail:
eBones project).
Sun SPOT developer's kit includes
- 2 x Full Sun SPOTs with eDemoSensor boards and batteries
- 1 x base-station Sun SPOT
- Software (Squawk VM, Java SDK, Netbeans)
- Other equipments (USB cable, Mounting clips, 2 x wall mounts, 1 x PC board mount)
Sun SPOT radio is based on IEEE 802.15.4 (Zigbee) that is lower cost and power but slower than Bluetooth (802.15.1) or Wi-Fi (802.11). The radio spec is 128-byte packets, ~70m range, 250Kbps, 2.4 GHz and "autonomous mesh networking" extends communication range. It also has easy interface with internet-connected devices via HTTP, TCP/IP* (*TCP/IP implementation is now on going).
The new feature of security aspect (for a forthcoming SDK release) is SSL protocol support. To support SSL, you can just change the current syntax for opening a radio stream connection:
conn = Connector.open(“radiostream://” + addr + “:” + port);
to
conn = Connector.open(“
sradiostream://” + addr + “:” + port);
Sun SPOT SDK also includes a great emulator, so you can try your program even if you don't have any real Sun SPOT!
In the field, Sun SPOT is utilized for several projects like "
Project Blackbox" to keep track of conditions present in the box while in transit and
Yggdrasil, common framework for data collection.
Project Live* [live-star]
Project Live* explores a new approach to software distribution and configuration of enterprise systems. Live* pushes the traditional installation process back to the factory. Software is distributed in the form of pre-installed immutable file system images that contain major software components, e.g. an OS, a database or an application server. At boot time Live* dynamically composes a collection of such modules into a single virtual software environment. Using a simple file system virtualization technology, Live* makes this composition entirely transparent to all existing software.
Also, similar to software in consumer electronics, Live* consolidates the system customization and configuration settings into a repository separate from the original software. This technology extends the concept of software distribution in the form of ready-to-run images like LiveCDs, with the true modularity and flexibility of customization.
In the demo room, the simplicity of Linux distribution upgrade is demonstrated as follows:
- A user runs a Red Hat version A (for Live*) on xVM
- the user makes a lot of customization on this system, e.g. network, font..
- the user upgrades OS to a new Red Hat version B (for Live*).
- After installation, he would usually need to reconfigure the above setting, but they are on Live* repository, so the user can use the new system seamlessly.
So, Live* software is even more advantageous when combined with VMware, xVM (Xen) or Virtual Box. It simplifies management while improving robustness and security.
Project SquawkSquawk is a Virtual Machine designed for resource constrained devices like Sun SPOT (and now being planned to port to LEGO Mindstorms NXT and Custom FPGA). The Squawk
- Implements J2ME CLDC 1.1/IMP 1.0 Profile
- Runs on the bare metal (No OS)
- Designed for memory constrained devices
- Written mainly in Java
- Runs multiple applications (isolates) that can be migrated across devices
- Also runs on Solaris, Linux, Mac and Windows
- Easy to port
Compared to Standard JVM, a lot of functions on Squawk JVM, e.g. loader, garbage collector, compiler and device driver, are written mainly in Java. With "isolates", One JVM OS process can run more than one applications on it and those applications can share some memories.